How to read a file with C#/.NET - Avoid the common mistakes
Time for another post in the series about solving common tasks with C#/.NET. For today's post, I'll dig into the challenge of reading a file. A lot of developers only know and use the File.ReadAllText
method, which is perfectly valid for small, short files. In this post, I'll show you the alternatives and list some of the pros and cons of the different possibilities.
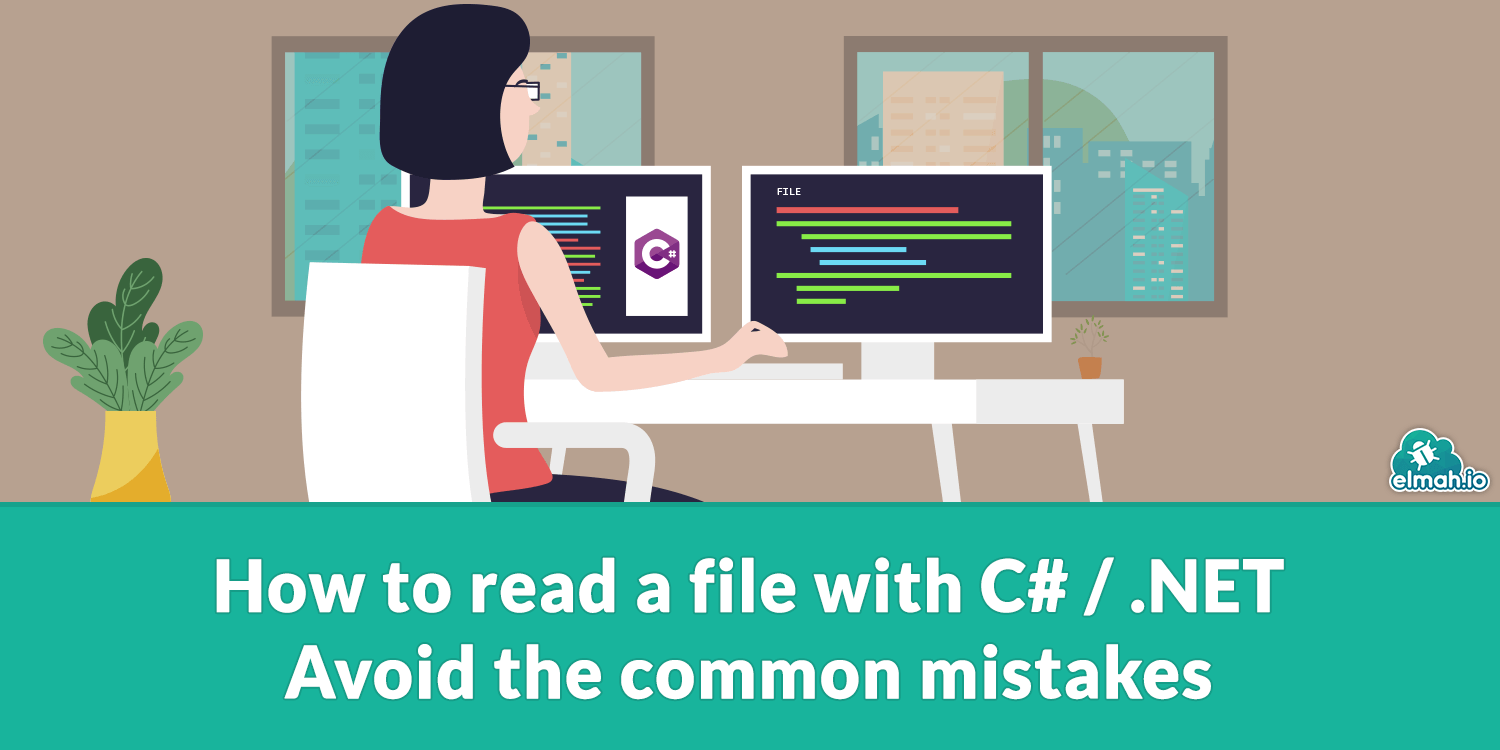
To read a file with C#, you can use the System.IO.File.ReadAllText
method, which allows you to read the contents of a text file into a string. The method takes the path of the file as an argument and returns the contents of the file as a string.
Here is an example of how to use the System.IO.File.ReadAllText
method to read the contents of a file:
string filePath = "C:\\myfolder\\myfile.txt";
string fileContents = System.IO.File.ReadAllText(filePath);
In this example, the filePath
variable contains the path to the file that you want to read and the fileContents
variable contains the contents of the file as a string.
There are a few common mistakes that people make when reading files with C#. Here are some tips to avoid these mistakes:
- Make sure that the file exists: Before reading a file, you should always check to make sure that the file exists at the specified path. If the file does not exist, the
System.IO.File.ReadAllText
method will throw aFileNotFoundException
error. To check if a file exists, you can use theSystem.IO.File.Exists
method, which returns abool
value indicating whether the file exists. - Handle exceptions gracefully: The
System.IO.File.ReadAllText
method can throw several different exceptions if there is an error while reading the file. For example, if the file is in use by another process, the method will throw anIOException
error. You should always use atry-catch
block to handle these exceptions gracefully and avoid crashing your application. - Use the correct file path: The file path that you provide to the
System.IO.File.ReadAllText
method should be the correct path to the file on the local file system. This means that the path should start with the drive letter and include the correct folder hierarchy and file name. You should also use the correct path separator for the operating system you are running on (forward slashes for Linux and Mac, and backslashes for Windows). - Close the file after reading it: After you have finished reading the contents of the file, you should always close the file to free up resources and prevent other processes from accessing the file. In C#, you can use the
using
statement to automatically close a file after it has been read. Theusing
statement ensures that the file is properly disposed of and released after it has been used.
Here is an example of how to read a file with C# while avoiding common mistakes:
string filePath = "C:\\myfolder\\myfile.txt";
try
{
// Check if the file exists
if (System.IO.File.Exists(filePath))
{
// Read the contents of the file
using (System.IO.StreamReader reader = System.IO.File.OpenText(filePath))
{
string fileContents = reader.ReadToEnd();
}
}
}
catch (Exception ex)
{
// Handle any exceptions that occurred
Console.WriteLine("An error occurred while reading the file: " + ex.Message);
}
In this example, the try-catch
block is used to handle any exceptions that might occur while reading the file.
elmah.io: Error logging and Uptime Monitoring for your web apps
This blog post is brought to you by elmah.io. elmah.io is error logging, uptime monitoring, deployment tracking, and service heartbeats for your .NET and JavaScript applications. Stop relying on your users to notify you when something is wrong or dig through hundreds of megabytes of log files spread across servers. With elmah.io, we store all of your log messages, notify you through popular channels like email, Slack, and Microsoft Teams, and help you fix errors fast.
See how we can help you monitor your website for crashes Monitor your website