How to map IPs to country for free with .NET and IP2Location 🌎
We do a lot of enrichments when processing log messages from our users. One of them is a mapping from an IP address to a location. We use a commercial database from IP2Location, but you can map IP addresses to location for free using their Lite dataset and .NET. In this post, I'll show you how to do that.
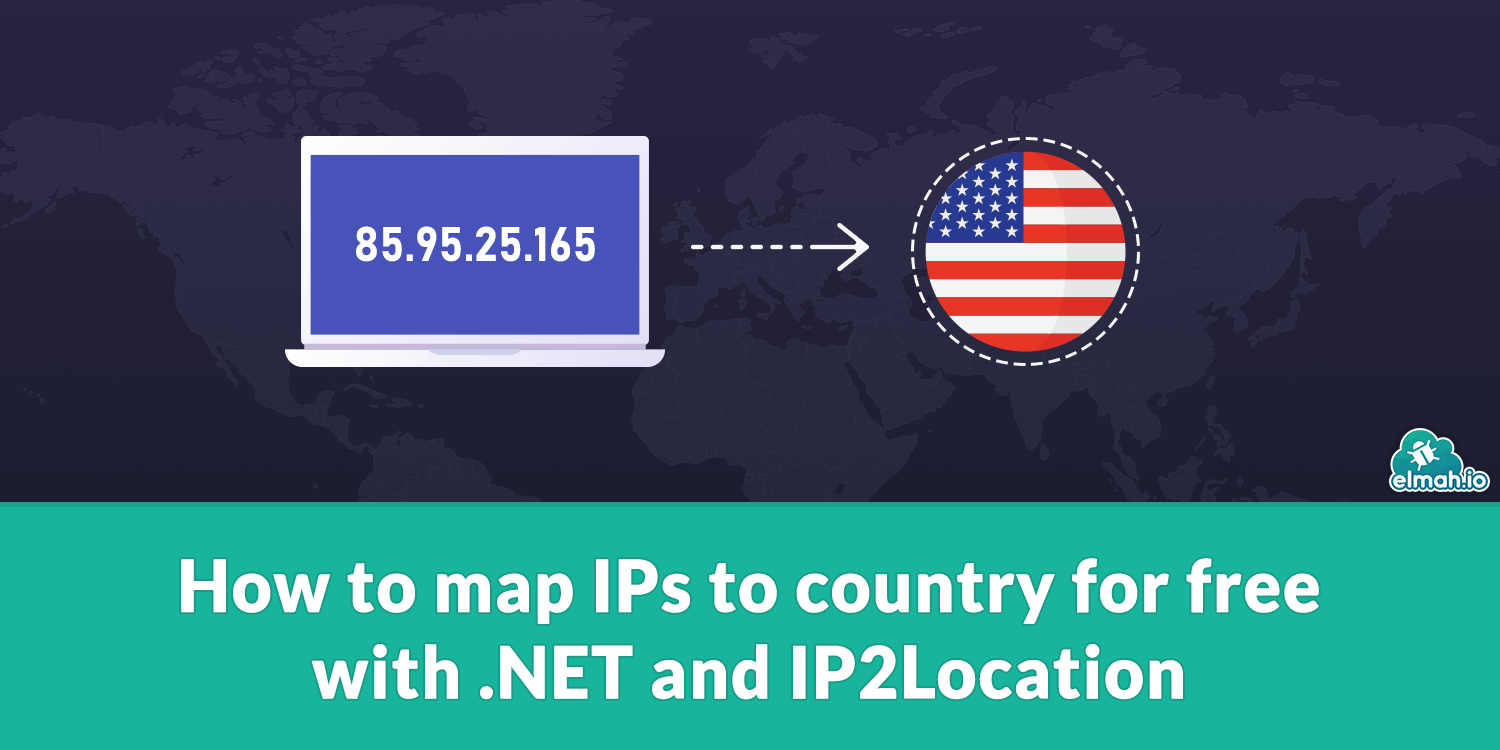
Before we begin, I want to put a few words on IP2Location. We are not affiliated with IP2Location in any way, and I only use that service because that is what we are using on elmah.io. There are a lot of both downloadable and REST-based IP-to-country resources and services out there. If you want a high match rate, you typically need to pay.
With that out of the way, let's start coding. For this blog post, I'll map an IP to a country, but there are options available for mapping to a city, latitude/longitude, etc. Start by downloading the IP2Location LITE Database from here: https://lite.ip2location.com/database/db1-ip-country. I will use the IPV4 BIN option. Next, create a new .NET Console application:
dotnet new console
Then, install the IP2Location.IPGeolocation
NuGet package:
dotnet add package IP2Location.IPGeolocation
Copy and paste the IP2LOCATION-LITE-DB1.BIN
file to the root of the new project and include it as part of the build output.
To map an IP address to a country, you will need an instance of the Component
class provided by the IP2Location.IPGeolocation
package:
Component ip2location = new Component
{
IPDatabasePath = "IP2LOCATION-LITE-DB1.BIN",
UseMemoryMappedFile = true,
};
In this example, the Component
class is created every time we run the console application. But if you are developing a long-running application like a Windows Service, you want to share the instance as a singleton. I have also set UseMemoryMappedFile
to true
to have IP2Location read the bin file into memory and creating lookups without having to touch the file.
Next, we will fetch a user-provided IP address and convert it to a country:
var ip = args[0];
var ipResult = ip2location.IPQuery(ip);
Console.WriteLine(ipResult.CountryLong);
Console.WriteLine(ipResult.CountryShort);
When running the code, the country's full name (CountryLong
) as well as the ISO Alpha-2 country code (CountryShort
):
United States of America
US
Mapping IP addresses to countries in .NET is surprisingly easy and completely free with IP2Location's LITE database. While the Lite version isn't as precise as the commercial options, it's a great starting point for enriching log data, providing geo-based analytics, or tailoring content based on location.
elmah.io: Error logging and Uptime Monitoring for your web apps
This blog post is brought to you by elmah.io. elmah.io is error logging, uptime monitoring, deployment tracking, and service heartbeats for your .NET and JavaScript applications. Stop relying on your users to notify you when something is wrong or dig through hundreds of megabytes of log files spread across servers. With elmah.io, we store all of your log messages, notify you through popular channels like email, Slack, and Microsoft Teams, and help you fix errors fast.
See how we can help you monitor your website for crashes Monitor your website