Easy generation of fake/dummy data in C# with Faker.Net 🕵️
When developing, testing, or showcasing something it's often important to use data that is not real data. Either because you might not have actual data available yet or because you don't want to show or pick the data from real users which can contain sensitive information. Faker.Net
can help make this possible by making it very simple to get a lot of random names, addresses, emails, etc. In this article, we will show the range of possible values it can create and how to use them.
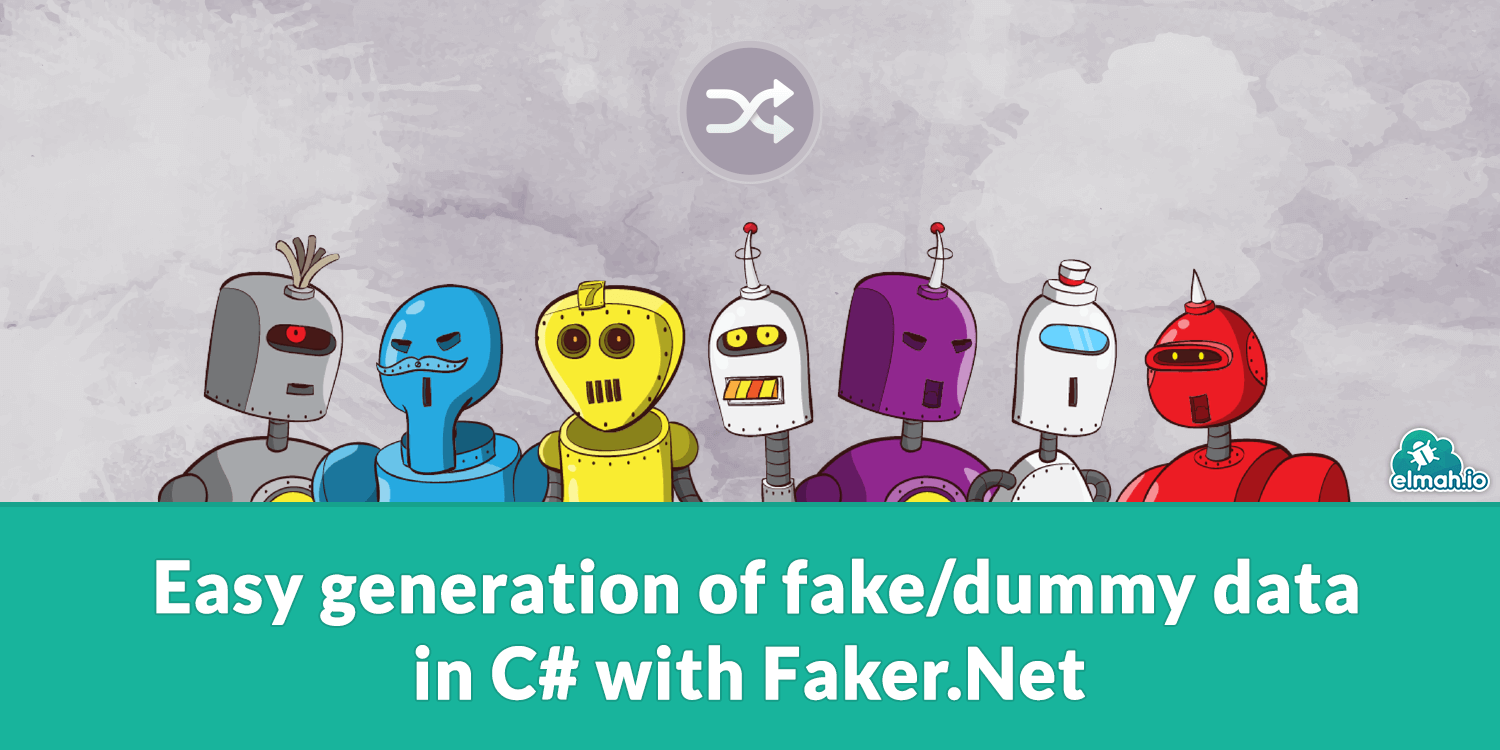
Quick history
The .NET library was initially a port of the 2009 version of the Ruby library Faker but has diverged in many ways. The Ruby library was itself a port of the Perl library Data::Faker from 2007. The library keeps being used and is updated to newer versions of .NET continuously. The cause for its continued use is probably due to its many use cases and very simple structure.
Getting started
First, we need to install the Faker.Net
package. We can do this through the Package Manager in Visual Studio or adding it through the CLI with:
dotnet add package Faker.Net
Now let's generate some data. We might want to create some instances of the following model.
class UserProfile
{
string Name { get; set; }
int Followers { get; set; }
string Area { get; set; }
string Bio { get; set; }
}
Here we need to generate a Name
, some random number in a plausible range, an Address
, and some random text for their Bio
.
We can generate a name from a combination of predefined First, Last, and Middle names together with optional suf/pre-fixes.
var user = new UserProfile();
user.Name = Faker.Name.FullName(NameFormats.WithPrefix);
// "Mrs. Jerod Nader"
In this case, we want the name to be specified with prefixes like "Mrs."
or "Mr."
. But we could also format the name ourselves by accessing the First
, Middle
, and Last
methods from Name
. Next, we want to generate a number for the Followers
field. We could have created a new Random
object instead and used its Next
method instead. Faker.Net
uses the RandomNumberGenerator
class from System.Security.Cryptography
instead, which can create even more random numbers and handles multithreading better.
user.Followers = Faker.RandomNumber.Next(0, 10000);
// 3452
Random numbers like these can be useful if you have some custom formatting for big numbers in your UI or just to see some varying data for a demo. Next, we want to generate where this user is from. For this, we want to get a random country and city.
user.Area = $"{Faker.Address.Country()}, {Faker.Address.City()}";
// "Fiji, Wavaland"
The country and city are generated independently so they will not fit together if you want to validate it later. You can also get Zipcodes and street names if you have a more complex address field. The last thing is to generate some text for the Bio
. We will simply use a simple Lorem Ipsum generator that is part of the library.
user.Bio = String.Join(" ", Faker.Lorem.Sentences(3));
// "Ea voluptas maiores nihil quia et eum. Vel et eos est architecto rerum est. Eum esse voluptatem ab necessitatibus."
Enums
We could imagine that we also had a field for the UserProfile
specifying their public visibility. This could be represented with an enum
like this.
enum Visibility
{
Public,
Private,
Internal,
}
Then we could get a random value for this enum
using Faker.Net
like so:
user.Visibility = Faker.Enum.Random<Visibility>();
// Visibility.Private
Domains and Emails
Domains and especially emails are also data types that are used a lot. This is one point where it is especially easy to see if you have simple used a random string or hardcoded a single email. We can generate a domain name like so:
user.CompanyDomain = Faker.Internet.DomainName();
// "torp.com"
And we can generate an email like so.
user.Email = Faker.Internet.Email(user.Name);
// "mrs_jerod.nader@hermanborer.name"
Here we used the user's previously set name for the email. It will internally use DomainName
to get a random domain for the email and convert the name to a username-style word. The DomainName
is picked from a list of plausible company names combined with a random valid top-level domain. We can also generate full URLs for something like user resources.
user.resources = Enumerable.Range(0,3).Select(_ => Faker.Internet.SecureUrl());
// "https://www.quigleyrobel.us/films/page.aspx"
// "https://www.braunborer.uk/guide/page.jsp"
// "https://www.jacobslind.biz/interviews/page.asp"
Conclusion
We have seen how we can use Faker.Net
to generate some random plausible data for some of the most used data types. This can make a big difference when you are testing or demoing a project both visually and functionally.
elmah.io: Error logging and Uptime Monitoring for your web apps
This blog post is brought to you by elmah.io. elmah.io is error logging, uptime monitoring, deployment tracking, and service heartbeats for your .NET and JavaScript applications. Stop relying on your users to notify you when something is wrong or dig through hundreds of megabytes of log files spread across servers. With elmah.io, we store all of your log messages, notify you through popular channels like email, Slack, and Microsoft Teams, and help you fix errors fast.
See how we can help you monitor your website for crashes Monitor your website