What's new in .NET 9: Cryptography improvements
.NET 9 is releasing in mid-November 2024. Like every .NET version, this introduces several important features and enhancements aligning developers with an ever-changing development ecosystem. In this blog series, I will explore critical updates in different areas of .NET. For today's post, I'll present some improvements to Cryptography.
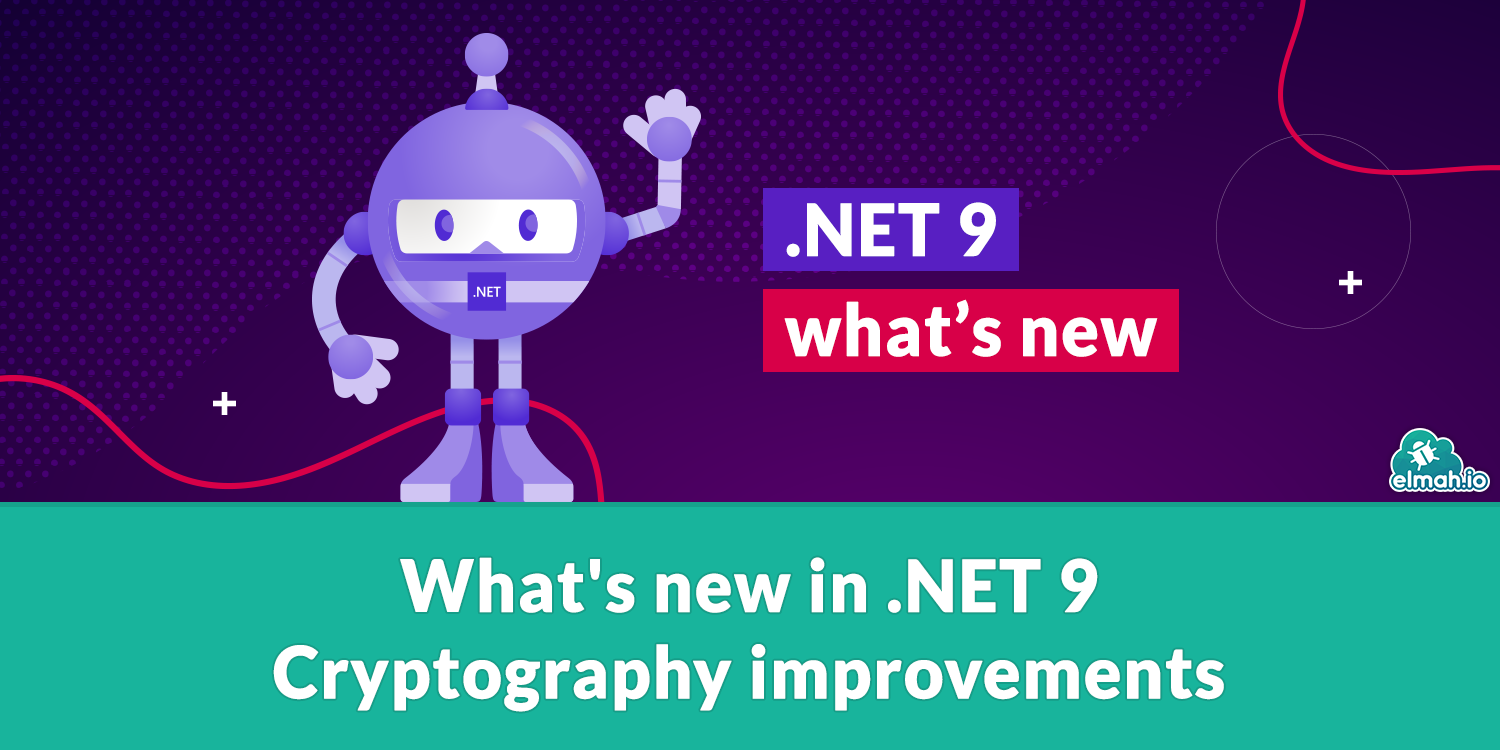
What is Cryptography in .NET
.NET provides a data-securing mechanism through encryption, decryption, hashing, and digital signature libraries. The System.Security.Cryptography
namespace contains classes that implement cryptography. It can be applied to any .NET application, such as a desktop app, Windows Presentation app, ASP.NET Core API, .NET MAUI, etc.
.NET 9 brings two novelties in cryptography.
CryptographicOperations.HashData
Traditionally, we have a memory-efficient and fast way to hash data. However, we have to provide a lot of code for different types of hashing algorithms. Changes in .NET 9 are succinctly doing this. CryptographicOperations.HashData
just takes the name of the hashing algorithm as a parameter and uses that to hash the input data. Unlike its preceding code, it does it much more concisely.
using System;
using System.Security.Cryptography;
using System.Text;
class Program
{
static void Main(string[] args)
{
// Data to hash
byte[] data = Encoding.UTF8.GetBytes("I am the string for hashing.");
string hashString = Convert.ToBase64String(hash);
Console.WriteLine($"Hash ({hash}): {hashString}");
}
}
So, what are some of the benefits of the new HashData
method? Besides improved performance by including this as a "one-shot" hashing method, code maintainability and flexibility are great advantages compared to previous versions of .NET. You can hash data simply by calling a single method and passing the desired hashing algorithm (e.g., SHA256, SHA512) as a parameter. The implementation makes code more readable, maintainable, and flexible by allowing you to switch algorithms.
KMAC (KECCAK Message Authentication Code)
KMAC adds security for data integrity. It ensures end-to-end security by its pseudorandom generation and authentication feature. KMAC has the following classes
Kmac128
: Generates 128-bit MACsKmac256
Generates 256-bit MACsKmacXof128
expandable output (max 128 bits at a time)KmacXof256
expandable output (max 256 bits at a time)
In the following code, we use it along with HashData
method on Kmax128
to secure the data:
using System.Security.Cryptography;
using System.Text;
class Program
{
static void Main(string[] args)
{
if (!Kmac128.IsSupported)
{
Console.WriteLine("KMAC is not supported on this system.");
return;
}
else
{
byte[] key = new byte[] { 1, 2, 3, 4, 5, 6, 7, 8, 9, 10, 11, 12, 13, 14, 15, 16 };
byte[] input = Encoding.UTF8.GetBytes("I am a string for hashing");
byte[] mac = Kmac128.HashData(key, input, outputLength: 32);
Console.WriteLine("KMAC (one-shot): {0}", Convert.ToBase64String(mac));
}
}
}
KMAC has minimum requirements for your system
- Linux with OpenSSL 3.0 or later.
- Windows system of Windows 11 Build 26016 or later.
Here are some of the benefits of using KMAC:
- Security Improvement: KMAC is a NIST SP800-185 algorithm trusted by many professionals. Its message authentication using pseudorandom generation adds higher security to the application.
- Flexible Output Length: KMACXof128 and KMACXof256 generate expandable output. Hence, KMAC is also suitable for applications requiring variable-length cryptographic output.
- System Compatibility Checks: The IsSupported property identifies KMAC's system compatibility and handles it before it leads to issues in production environments when running on unsupported platforms.
- One-Shot and Accumulation Modes: KMAC allows developers to either use a one-shot method (Kmac128.HashData) for quick operations or accumulate data in parts to calculate a MAC, providing flexibility based on different use cases.
- Latest system compatibility: KMAC especially supports the latest versions of Windows and Unix-based systems, aligning your application to modern security standards.
Conclusion
.NET 9 was released and brought many improvements in the development ecosystem. In this post, I discussed additions and updates in cryptography in .NET 9. CryptographicOperations.HashData
and KMAC are additions to the Cryptography namespace. HashData
encloses hashing algorithm selection inside the method. You only specify the algorithm to use. KMAC is a secure NIST SP-800–185 end-to-end encryption method that you can use in .NET 9 applications and forward.
elmah.io: Error logging and Uptime Monitoring for your web apps
This blog post is brought to you by elmah.io. elmah.io is error logging, uptime monitoring, deployment tracking, and service heartbeats for your .NET and JavaScript applications. Stop relying on your users to notify you when something is wrong or dig through hundreds of megabytes of log files spread across servers. With elmah.io, we store all of your log messages, notify you through popular channels like email, Slack, and Microsoft Teams, and help you fix errors fast.
See how we can help you monitor your website for crashes Monitor your website