Validate JSON files against schema in Azure DevOps build
JSON files have become part of our daily lives. We use JSON files for all sorts of tasks like settings, defining database schemas, and much more. The other day I found out that invalid JSON files had been pushed to one of our repositories. So, I decided to include JSON file validation as part of our build on Azure DevOps. In this post, I'll share the solution.
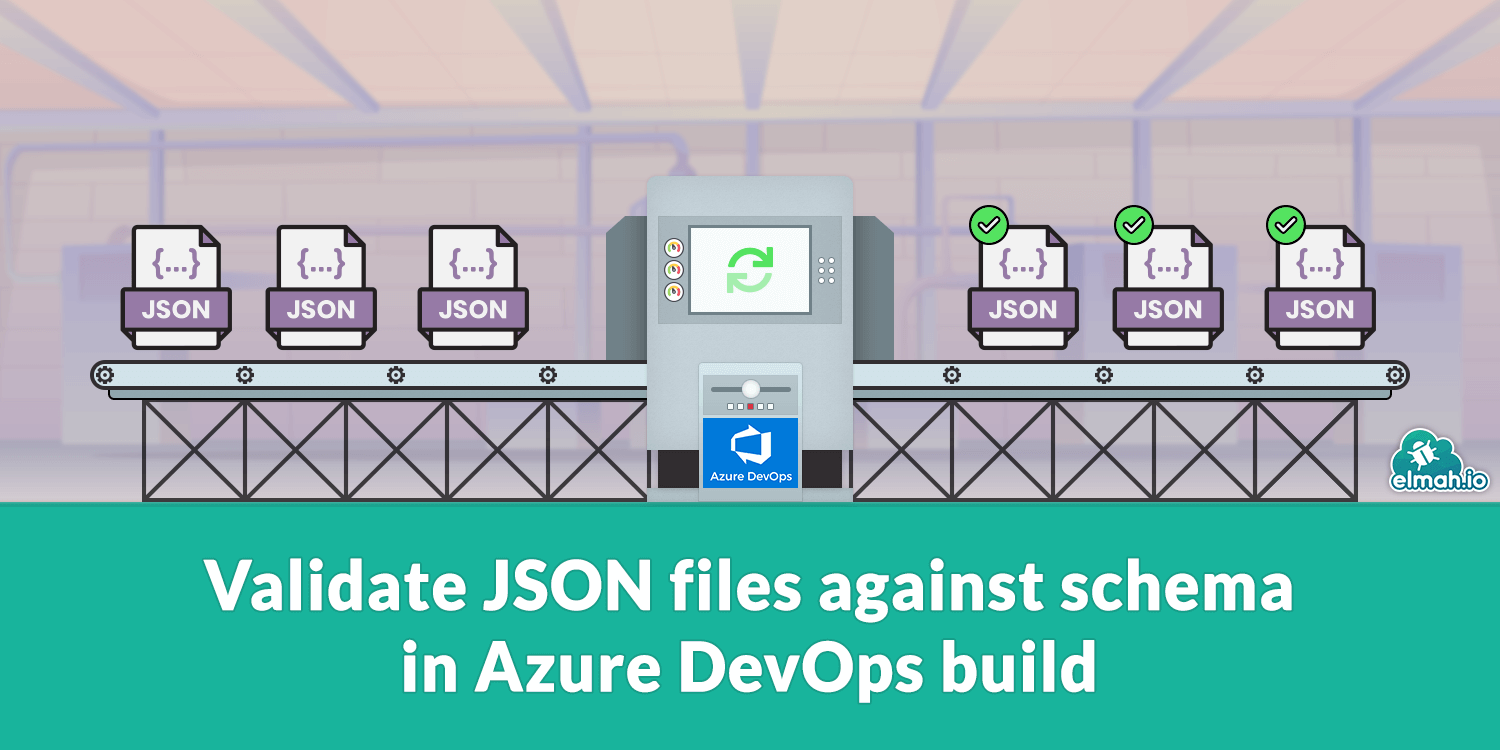
I'm sure you can think of a scenario where invalid JSON files either do not parse as valid syntax or don't conform to the intended format. Think of an invalid JSON configuration file being shipped to your ASP.NET Core website on production. Result: the website crashes.
Let's build a simple JSON file validation step as part of a pipeline on Azure DevOps. This post will include a solution for both the classic editor as well as the YAML-based pipeline. The steps can be easily duplicated to GitHub Actions and other build servers since they don't use any DevOps-specific steps or extensions.
For this post, I have created a git repository on Azure DevOps with two files: file.json
and schema.json
. These are imaginary files representing a JSON file in your code and a JSON Schema file. If you prefer only to validate the JSON syntax you don't need the schema.json
file, but I like validating both syntax and content.
Let's start with the classic editor. Add a new build step and pick the PowerShell Script task:
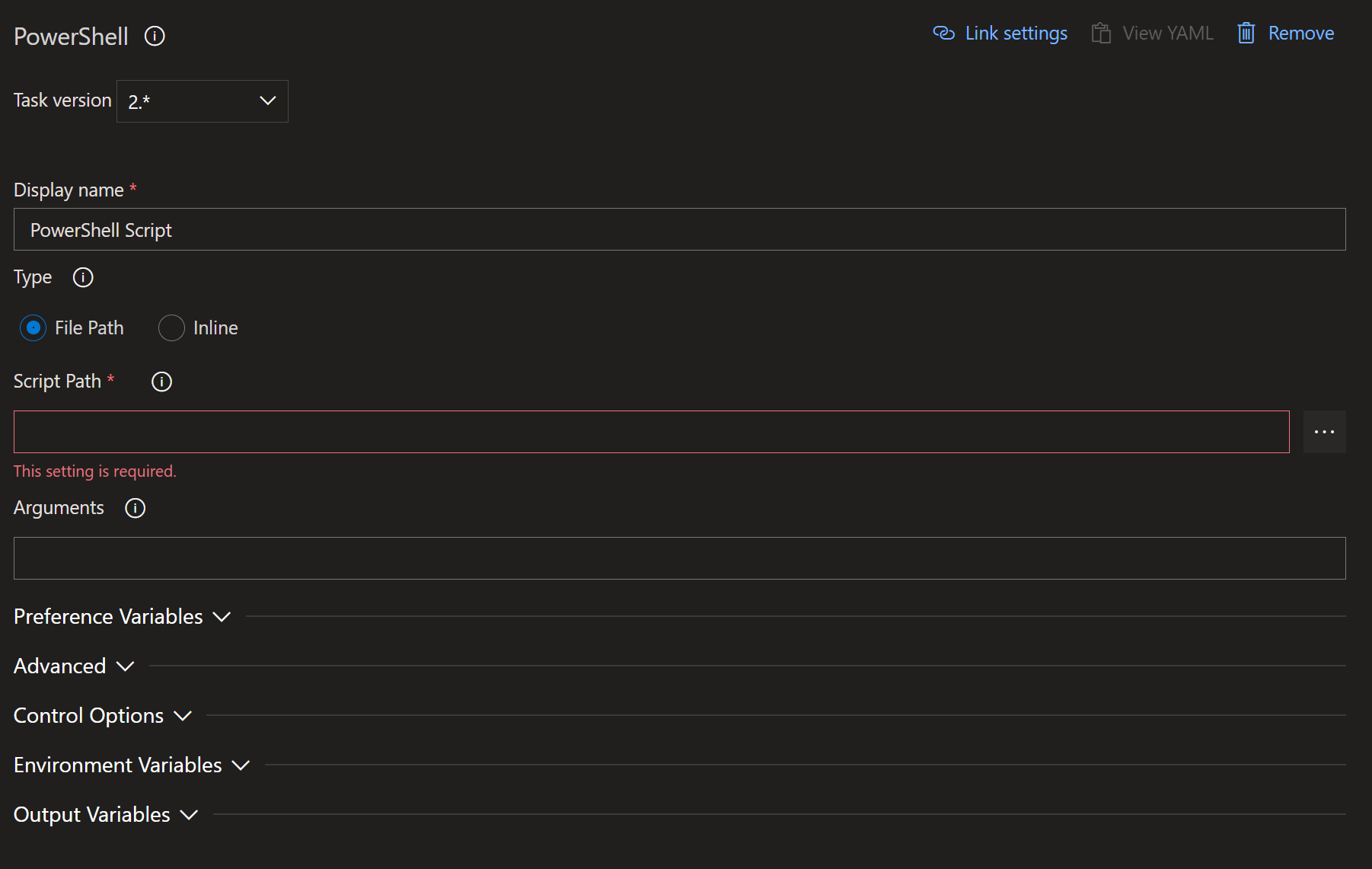
In Type select Inline and copy/paste the following PowerShell code:
[array]$jsonFiles=Get-ChildItem .\*.json -exclude schema.json | select -expand fullname
foreach ($filePath in $jsonFiles)
{
Write-Host "Validating $filePath"
Get-Content $filePath -Raw | Test-Json -SchemaFile .\schema.json
}
This will select a list of all .json
files (except the schema.json
file) and run the Test-Json
Cmdlet on each file. Test-Json
is part of PowerShell newer than version 5 which is used as a default on Azure DevOps. To get the most recent PowerShell version, make sure to check the Use PowerShell Core checkbox beneath Advanced:

When running the build, you should see the JSON file in the repository being successfully validated:
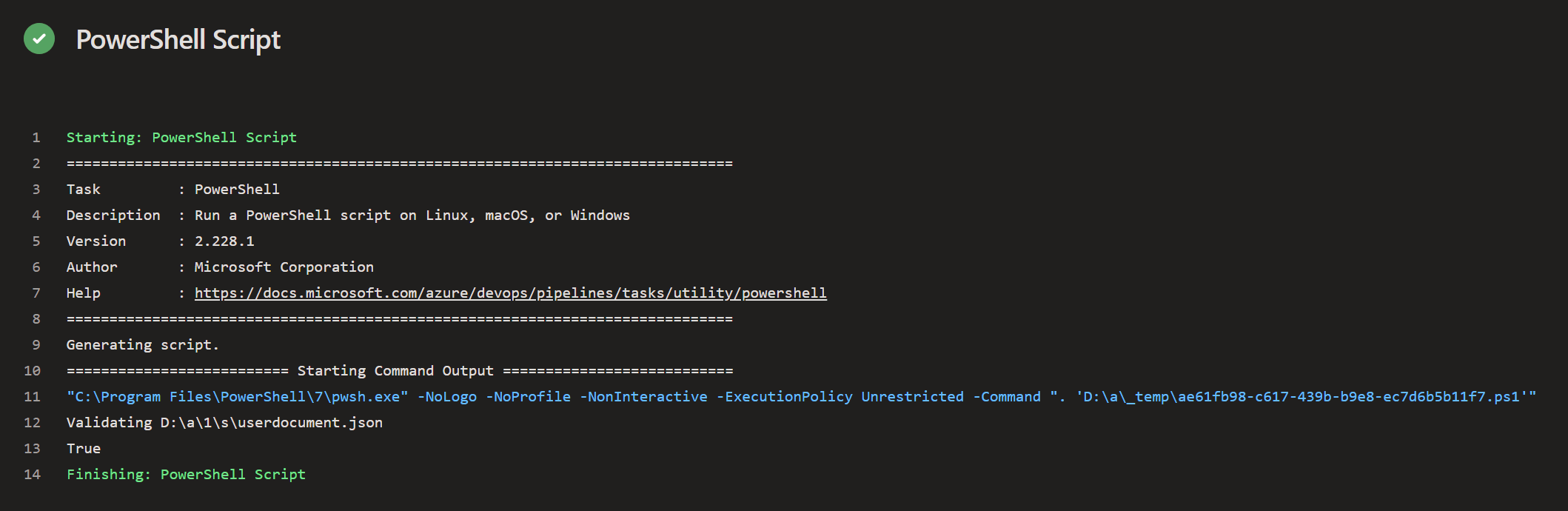
The Test-Json
Cmdlet outputs a boolean indicating the status. In case this boolean is False
the build will fail.
The same task can be included in a YAML-based build pipeline by including this build step:
trigger:
- master
pool:
vmImage: ubuntu-latest
steps:
- task: PowerShell@2
inputs:
targetType: 'inline'
script: |
[array]$jsonFiles=Get-ChildItem .\*.json -exclude schema.json | select -expand fullname
foreach ($filePath in $jsonFiles)
{
Write-Host "Validating $filePath"
Get-Content $filePath -Raw | Test-Json -SchemaFile .\schema.json
}
The PowerShell code should look familiar from the previous example. The build uses Ubuntu as the VM image which comes with PowerShell 7 out of the box. If you are using windows-latest
you will have the same problem with PowerShell 5 as in the classic example. In this case, you'd want to set pwsh
to true
:
trigger:
- master
pool:
vmImage: windows-latest
steps:
- task: PowerShell@2
inputs:
targetType: 'inline'
pwsh: true
script: |
[array]$jsonFiles=Get-ChildItem .\*.json -exclude schema.json | select -expand fullname
foreach ($filePath in $jsonFiles)
{
Write-Host "Validating $filePath"
Get-Content $filePath -Raw | Test-Json -SchemaFile .\schema.json
}
elmah.io: Error logging and Uptime Monitoring for your web apps
This blog post is brought to you by elmah.io. elmah.io is error logging, uptime monitoring, deployment tracking, and service heartbeats for your .NET and JavaScript applications. Stop relying on your users to notify you when something is wrong or dig through hundreds of megabytes of log files spread across servers. With elmah.io, we store all of your log messages, notify you through popular channels like email, Slack, and Microsoft Teams, and help you fix errors fast.
See how we can help you monitor your website for crashes Monitor your website