Leveraging Tuples in C#: Real-World Use Cases
Well, using OOP in development is a blessing. We define classes to leverage inheritance and abstraction. We easily represent one object as a model. However, using and maintaining these classes can sometimes be tedious for the developers and the application’s memory. You might need to define separate classes or structs for each data collection passed in and out of methods. Today, I will introduce you to C# tuple, a solution for this problem that avoids creating separate classes for intermediate data representations. We will see how you can define a tuple for several scenarios and reduce memory load and unnecessary work.
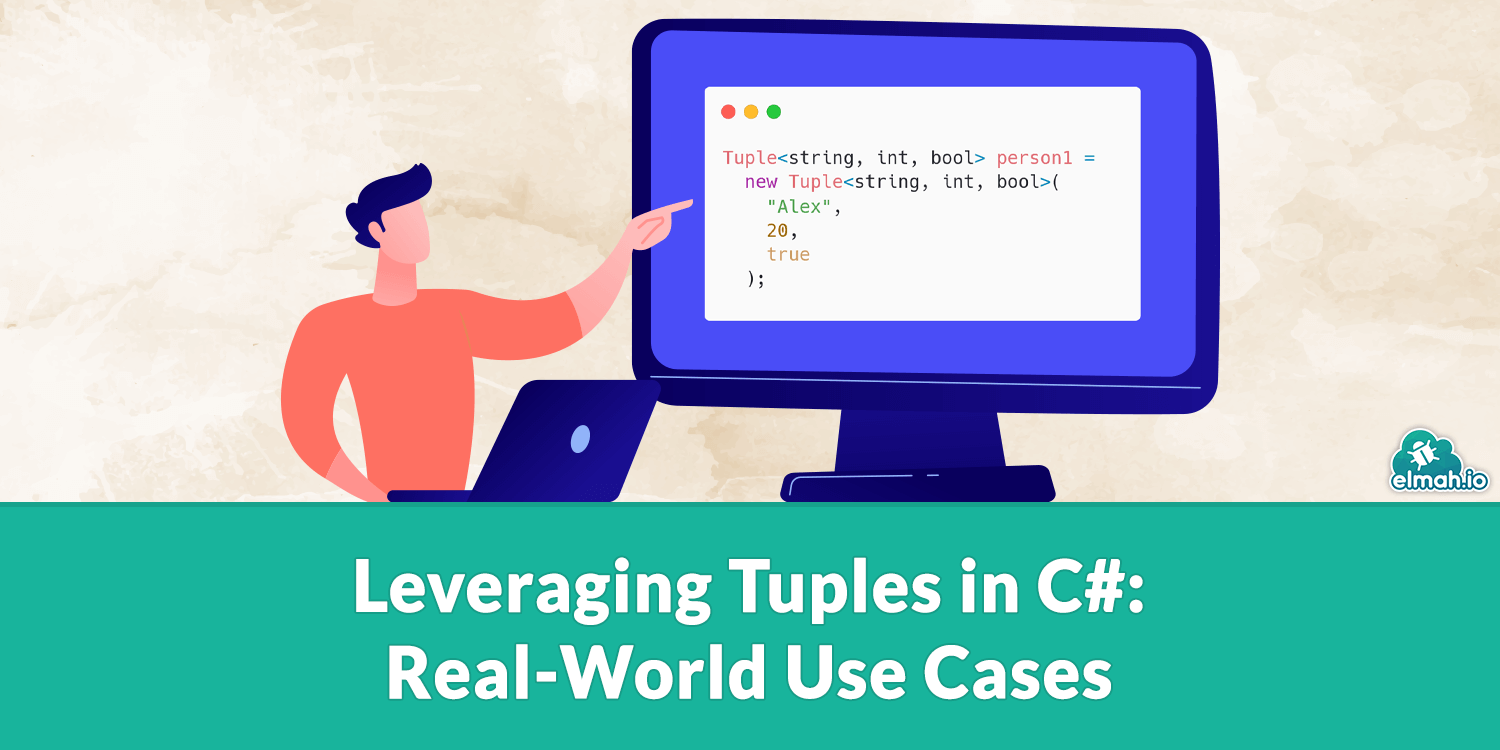
What is Tuple in C#?
A tuple is a lightweight, immutable data structure with a collection of values. Like classes, it allows storing and representing multiple fields within a single entity. However, tuples are more concise and do not require a predefined blueprint, making them both memory- and time-efficient.
Tuples are particularly useful when you need to return multiple values from a method without the overhead of declaring a separate class or struct. They provide a quick and simple way to group related data while keeping your code clean and efficient.
Let's move towards the coding implementation of a tuple. We can define tuples in different ways:
// Using Tuple class
Tuple<string, int, bool> person1 = new Tuple<string, int, bool>("Alex", 20, true);
With the newer version of C#, you can do it more concisely and cleanly:
// Using tuple literal syntax (C# 7.0 and later)
var person2 = ("Trevis", 26, true);
Also, you can explicitly name the fields:
(string Name, int Age, bool IsActive) person3 = ("Jonathan", 23,true);
If fields are not named explicitly like the first 2 examples, we can access these fields as Item1
and Item2
:
// Printing person1
Console.WriteLine(person1.Item1);
Console.WriteLine(person1.Item2);
Console.WriteLine(person1.Item3);
// Printing person2
Console.WriteLine(person2.Item1);
Console.WriteLine(person2.Item2);
Console.WriteLine(person2.Item3);
Output
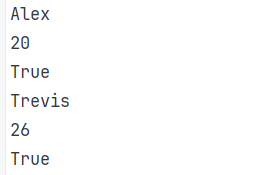
While with the 3rd example:
// Printing person3
Console.WriteLine(person3.Name);
Console.WriteLine(person3.Age);
Console.WriteLine(person3.IsActive);
Output
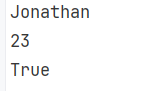
Or you can print the whole tuple:
Console.WriteLine(person3);
Output

Nested Tuple
You can create tuples inside tuples, forming nested tuples:
var employees = (("Younus",33), ("Dhoni",45), ("Tom", 37));
Console.WriteLine(employees.Item1);
Console.WriteLine(employees.Item1.Item1);
Output
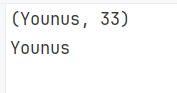
Tuple Deconstruction in C#
You can get the fields of a tuple in a more readable way by deconstructing them:
var location = (Latitude: 49.0232, Longitude: -98.4822);
// Deconstruct tuple to get its fields as variables
var (lat, lon) = location;
Console.WriteLine($"Latitude: {lat}, Longitude: {lon}");
Output

We have seen how to define tuples. Now, let's dive through some real examples of usage.
Example 1: Tuple as a function parameter in C#
Passing a tuple is a good idea when you have an intermediate collection of values and are not passing any database model or input DTOs. You do not need to define a separate class or structure for one-time use:
void DisplayLocation((double Latitude, double Longitude) location)
{
Console.WriteLine($"Latitude: {location.Latitude}, Longitude: {location.Longitude}");
}
// Example usage
var location = (Latitude: 49.0232, Longitude: -98.4822);
DisplayLocation(location);
Output

Example 2: Tuple as the return type of a method in C#
For example, you are building an email message based on the email type in a method. So, you will return two values, subject and body, which you can simply consolidate as a tuple. Using a tuple as an email message is a widely used scenario.
Step 1: define an EmailType
enum
public enum EmailTypeEnum
{
WelcomeEmail,
PasswordReset,
SubscriptionRenewal
}
Step 2: define method
// Method to generate the subject and body of the email
(string Subject, string Body) GenerateEmailContent(EmailTypeEnum emailType, string recipientName)
{
switch (emailType)
{
case EmailTypeEnum.WelcomeEmail:
return ($"Welcome to Our Platform, {recipientName}!",
$"Hi {recipientName}, welcome aboard! We're excited to have you.");
case EmailTypeEnum.PasswordReset:
return ($"Password Reset Request",
$"Hi {recipientName}, click the link to reset your password.");
case EmailTypeEnum.SubscriptionRenewal:
return ($"Subscription Renewal Reminder",
$"Hi {recipientName}, renew your subscription to keep enjoying our service.");
default:
return ("Unknown Email Type", "No content available.");
}
}
Step 3: Call the method
var emailContent1 = GenerateEmailContent(EmailTypeEnum.WelcomeEmail, "Thomas");
Console.WriteLine($"Subject: {emailContent1.Subject}");
Console.WriteLine($"Body: {emailContent1.Body}");
var emailContent2 = GenerateEmailContent(EmailTypeEnum.SubscriptionRenewal, "Daniel");
Console.WriteLine($"Subject: {emailContent2.Subject}");
Console.WriteLine($"Body: {emailContent2.Body}");
Output

Why use C# tuples?
Tuples are useful in reducing complexity and streamlining the code. First, they save extra work and unnecessary memory by creating a class. Second, a Tuple allows for the concise return of multiple values. You can destructure them directly, making them readable compared to other data structure options. Finally, the code looks clearer by eliminating such intermediate one-time classes, improving readability and maintainability.
Best Practices
Tuples can help more if you utilize its potential with your application requirements. Consider tuples for small data collections where a class model is unnecessary while using tuples for a complex representation can lead to maintainability and readability issues. Provide meaningful names for tuple fields to avoid any ambiguity while using those fields. You should analyze the usability of the scenario before employing tuples. In some cases, other options like struct, records, and class are better than tuples.
Conclusion
Tuple is a C# data structure that provides a lightweight, immutable solution for storing and representing a collection of values. It is a flexible alternative to class and struct in scenarios where a collection of values is required for intermediate use, such as a function's input or return type. By using tuples with best practices, you can avoid unnecessary code and leverage with its concise and readable code.
elmah.io: Error logging and Uptime Monitoring for your web apps
This blog post is brought to you by elmah.io. elmah.io is error logging, uptime monitoring, deployment tracking, and service heartbeats for your .NET and JavaScript applications. Stop relying on your users to notify you when something is wrong or dig through hundreds of megabytes of log files spread across servers. With elmah.io, we store all of your log messages, notify you through popular channels like email, Slack, and Microsoft Teams, and help you fix errors fast.
See how we can help you monitor your website for crashes Monitor your website