Individual developer settings in ASP.NET Core
So, you started using ASP.NET Core and love the new hierarchical JSON-based settings. But now you realize that you need individual settings per developer on your team. In this post, I will show you the best way to achieve just that.
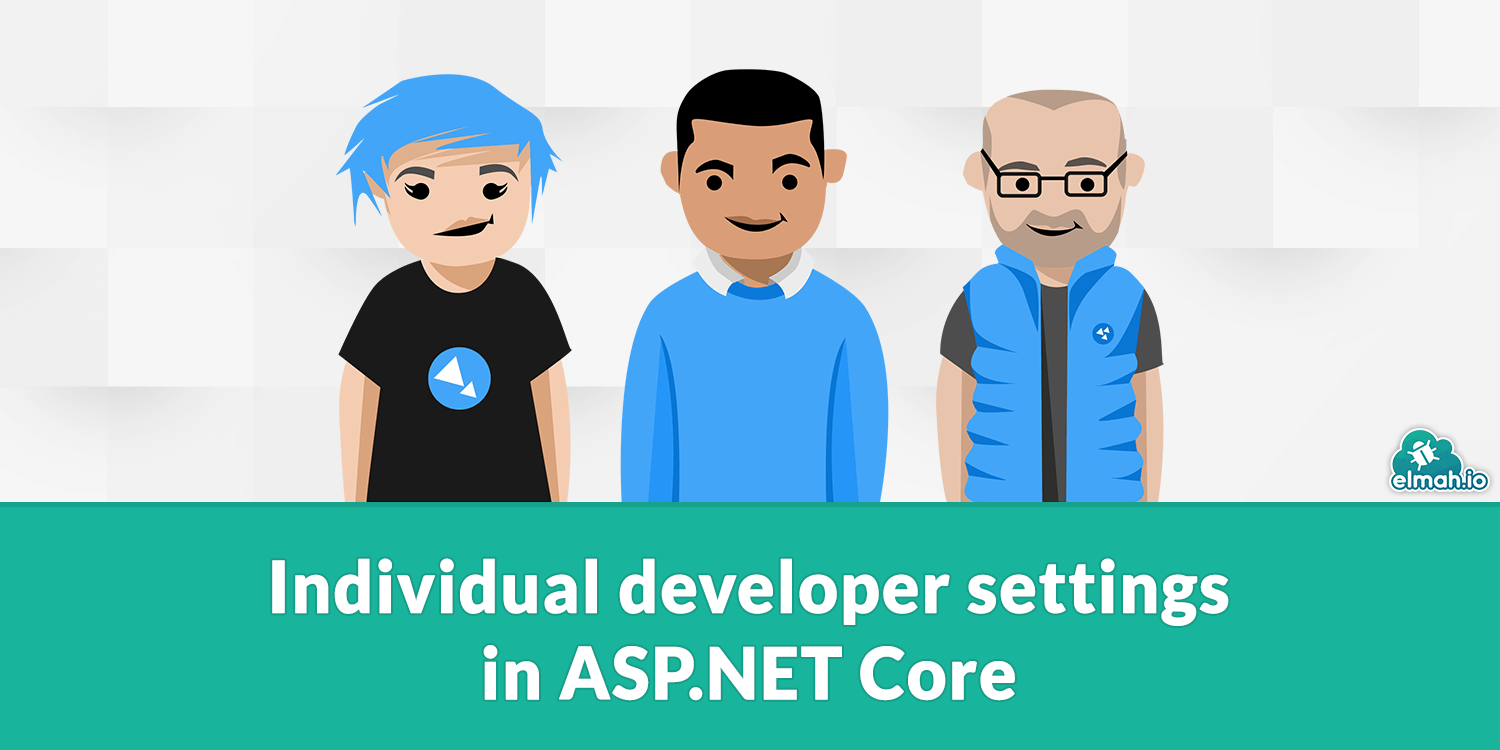
Let's start by looking at settings in ASP.NET Core. You may remember <AppSettings>
from .NET Full, and luckily, ASP.NET Core (and .NET Core) have a similar construct. In short, you create a new file named appsettings.json
and start adding application settings as JSON properties. The file is simply a JSON file which you have awesome tool support for with Visual Studio and Code and you can validate and pretty print it with this JSON formatter and validator. For the full picture, check out this blog post: AppSettings in ASP.NET Core.
A valid appsettings.json
file could look like this:
{
"AppSettings": {
"ConnectionString": "http://localhost:9000"
},
...
}
In there, I specified a new object named AppSettings
including a single property name ConnectionString
with the value of http://localhost:9000
. Getting the value from code can either use the full path:
IConfiguration config = ...;
var value = config["AppSettings:ConnectionString"]
Or you can create a strongly typed object representing the AppSettings
JSON-object:
public class AppSettings
{
public string ConnectionString { get; set; }
}
services.Configure<AppSettings>(appSettings);
By calling the Configure
-method, you can use dependency injection in ASP.NET Core to inject an AppSettings
object into your code.
You now have a hard-coded connection string in your source code, which will end up in a source control system. But what if your code is dependent on a central database with individual users for your developers? The connection string could look something like this:
http://localhost:9000?user=richard&password=1234
The connection string doesn't match a specific database technology, but I'm sure you get the point. The connection string is hard-coded to the user Richard. When Dinesh wants to clone the code and starts developing, he needs to change the setting in appsettings.json
.
Environment variables to the rescue. Application settings in .NET Core play very well with environment variables. You can right-click the project, click Properties, select the Debug tab and input a new variable beneath Environment variables:
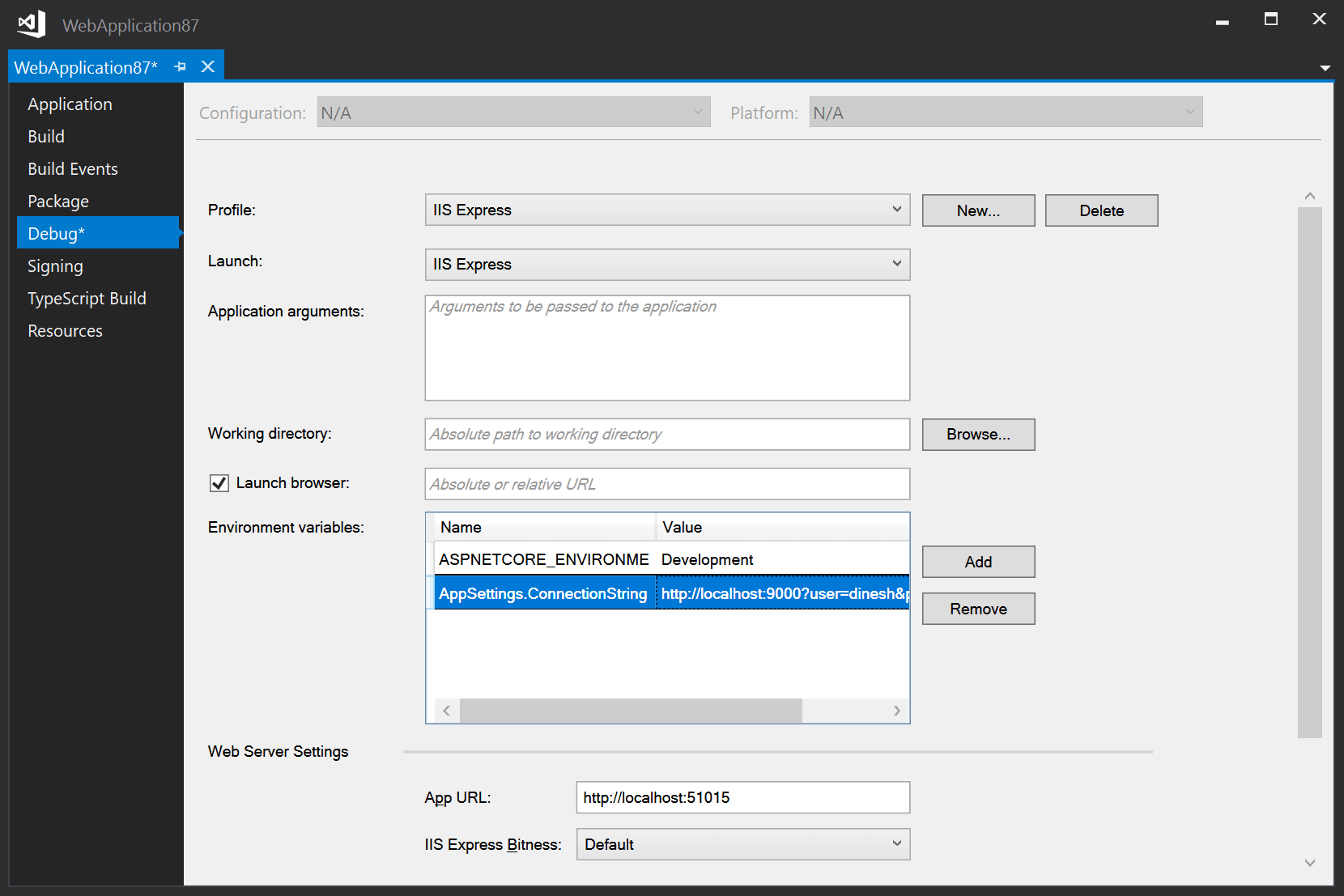
Notice that the full path is specified with a comma: AppSettings:ConnectionString
.
The variable is stored in the launchSettings.json
file, located in the Properties
folder. This file is under normal circumstances not pushed to source control. In case you want to re-use the key across multiple projects, you can use environment variables built into Windows:
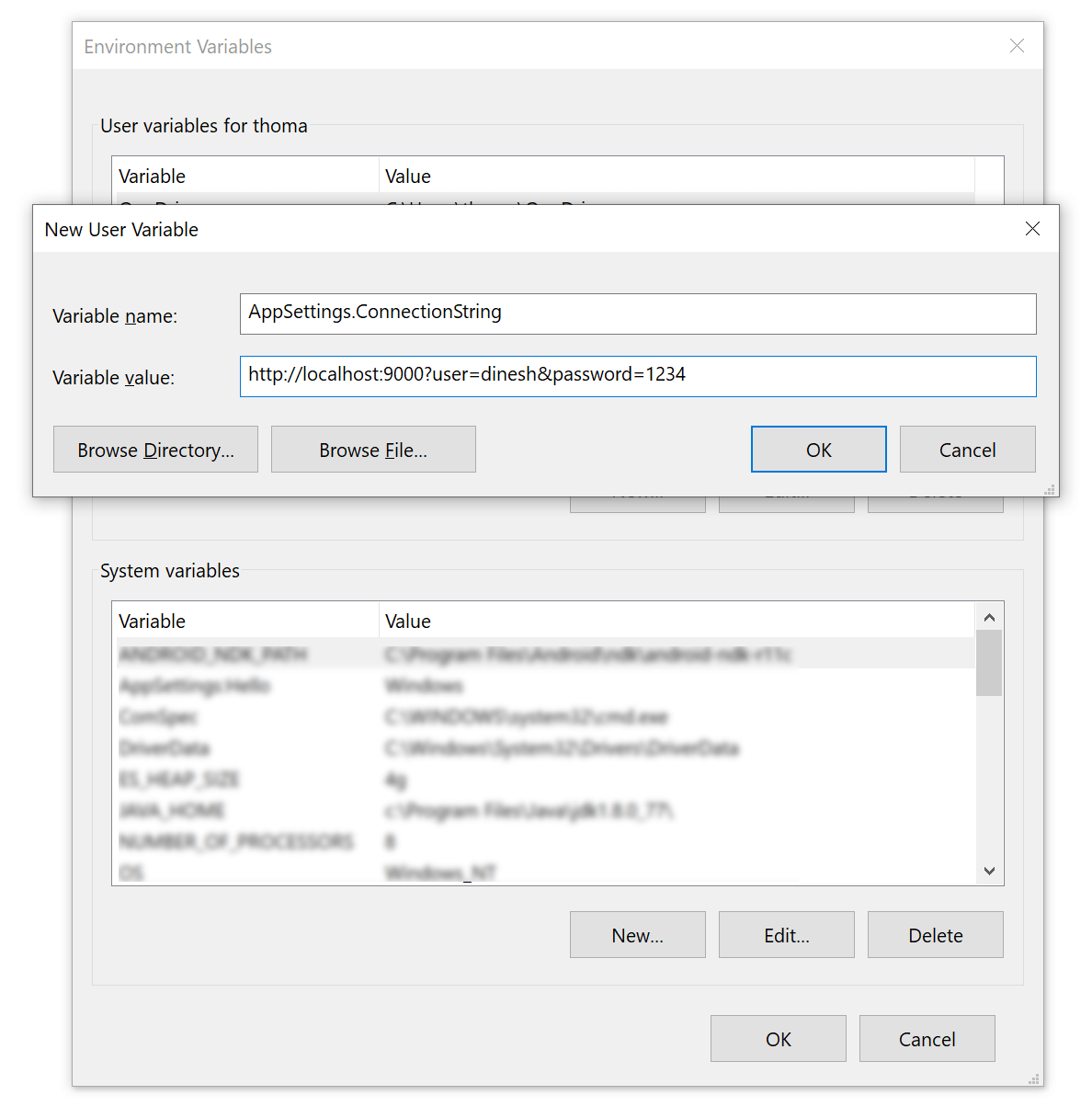
If you are calling the CreateDefaultBuilder
-method in your Program.cs
file, you are ready to click F5 and launch your project. If not, make sure to call the AddEnvironmentVariables
-method:
public static IWebHostBuilder CreateWebHostBuilder(string[] args) =>
WebHost.CreateDefaultBuilder(args)
.ConfigureAppConfiguration((ctx, builder) =>
{
...
builder.AddEnvironmentVariables();
})
.UseStartup<Startup>();
If you still want developer settings to be pushed to source control, you can utilize the flexibility of the configuration system in ASP.NET Core, by creating a configuration file per developer machine:
{
"AppSettings": {
"ConnectionString": "http://localhost:9000?user=gilfoyle&password=5678"
}
}
Name this file as the machine it should work on (like appsettings.gilfoyle.json
) and make sure to add the following to the Program.cs
file:
WebHost.CreateDefaultBuilder(args)
.ConfigureAppConfiguration((ctx, builder) =>
{
...
builder.AddJsonFile("appsettings.json", false, true);
builder.AddJsonFile($"appsettings.{Environment.MachineName}.json", true, true);
})
.UseStartup<Startup>();
In the example, I tell ASP.NET Core to read settings from the appsettings.json
file and override it with any settings in the appsettings.{Environment.MachineName}.json
file where {Environment.MachineName}
is replaced with the actual machine name on runtime. This way, you can specify an override file per machine. Make sure that each override file works as you'd expect by validating it using this Appsettings.json Transformation Tester.
This post showed you how to specify settings per developer. While the proposed solutions can be excellent for non-secure settings, I don't recommend you to add connection strings and similar to source control. ASP.NET Core offers a feature to overcome this called user secrets. To learn more about this feature, check out our blog post ASP.NET Core (not that secret) User Secrets Explained.
elmah.io: Error logging and Uptime Monitoring for your web apps
This blog post is brought to you by elmah.io. elmah.io is error logging, uptime monitoring, deployment tracking, and service heartbeats for your .NET and JavaScript applications. Stop relying on your users to notify you when something is wrong or dig through hundreds of megabytes of log files spread across servers. With elmah.io, we store all of your log messages, notify you through popular channels like email, Slack, and Microsoft Teams, and help you fix errors fast.
See how we can help you monitor your website for crashes Monitor your website