Effective Error Logging in Windows Forms Applications with C#
Error logging is an essential part of any application, as it allows developers to track and fix issues that may arise during the use of the application. In a Windows Forms application written in C#, several options exist for implementing effective error logging. In this blog post, we will discuss the various approaches for logging errors in a Windows Forms application, and provide guidance on the best practices for implementing error logging in your own applications.
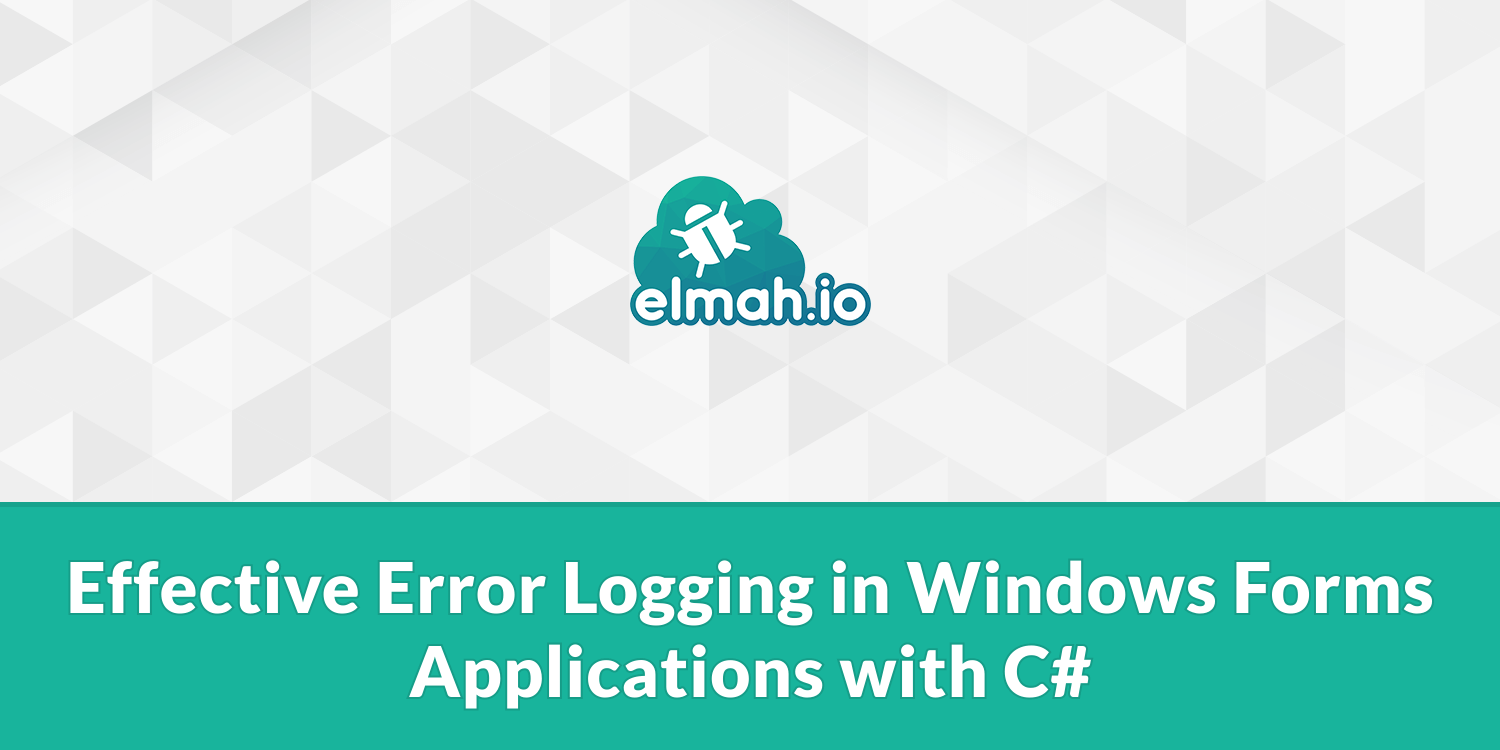
I'll use a simple file as an output to keep the examples simple. In a real application, you'd want to use a logging framework like Serilog or NLog (maybe even log errors to elmah.io).
Use try/catch blocks to handle exceptions
One of the most basic ways to log errors in a Windows Forms application is to use try/catch blocks to handle exceptions that may occur. When an exception is thrown, the catch block can log the error to a file or database, and then display a user-friendly message to the user. This approach is useful for catching and handling specific exceptions that you anticipate may occur in your application.
Here is an example of using try/catch
blocks to handle exceptions:
try
{
// Code that may throw an exception
}
catch (Exception ex)
{
// Log the error
File.AppendAllText("error.log", ex.ToString());
// Display user-friendly message
MessageBox.Show("An error has occurred. Please try again later.");
}
It is important to note that try/catch
blocks should only be used to handle exceptions that you anticipate may occur and that you have the ability to recover from. For example, if you are parsing user input and expect that the input may not always be in the correct format, you can use a try/catch
block to catch the exception and display a user-friendly message to the user. When possible, always try to avoid exceptions as shown in this post: C# exception handling best practices.
Use the Application.ThreadException event
The Application.ThreadException
event allows you to handle unhandled exceptions that occur on the UI thread. This is useful for catching any exceptions that may not be handled by try/catch
blocks, and allows you to log the error and display a user-friendly message to the user.
Here is an example of using the Application.ThreadException
event to handle unhandled exceptions:
private void Application_ThreadException(object sender, ThreadExceptionEventArgs e)
{
// Log the error
File.AppendAllText("error.log", e.Exception.ToString());
// Display user-friendly message
MessageBox.Show("An error has occurred. Please try again later.");
}
// Register the event handler
Application.ThreadException += new ThreadExceptionEventHandler(Application_ThreadException);
It is important to note that the Application.ThreadException
event should only be used to handle unhandled exceptions on the UI thread. If an exception occurs on a non-UI thread, it will not be caught by this event.
Use a global exception handler
Another option for logging errors in a Windows Forms application is to use a global exception handler. This involves creating a custom class that implements the UnhandledException
event and registers it as the global exception handler for the application. This allows you to catch and handle any unhandled exceptions that may occur in your application, and log the error for future debugging.
Here is an example of using a global exception handler to log errors:
public class GlobalExceptionHandler
{
public static void UnhandledException(object sender, UnhandledExceptionEventArgs e)
{
// Log the error
File.AppendAllText("error.log", e.ExceptionObject.ToString());
// Display user-friendly message
MessageBox.Show("An error has occurred. Please try again later.");
}
}
// Register the global exception handler
AppDomain.CurrentDomain.UnhandledException += new UnhandledExceptionEventHandler(GlobalExceptionHandler.UnhandledException);
The global exception handler is useful for catching any unhandled exceptions that may not be caught by try/catch
blocks or the Application.ThreadException
event. It is important to note that the global exception handler should only be used as a last resort, as it will catch all unhandled exceptions in the application. You need to include it, though, to make sure that all exceptions are correctly handled. Even the ones you didn't expect.
Best practices
- Use multiple approaches for logging errors: It is recommended to use a combination of
try/catch
blocks, theApplication.ThreadException
event, and a global exception handler to ensure that all errors are logged and handled in your application. - Log as much information as possible: To properly debug and fix errors, it is important to log as much information as possible about the error, such as the exception type, message, and stack trace. When using a service like elmah.io together with a logging framework, we automatically pick up all of the needed information to inspect what went wrong.
- Display user-friendly messages: When an error occurs, it is important to display a user-friendly message to the user, rather than displaying a technical error message. This helps to improve the user experience and prevent confusion.
- Handle errors on non-UI threads: If your application has background tasks or worker threads that perform operations, it is also important to handle any errors that may occur on these threads. One way to do this is to use the
Task.Run
method and specify a catch block to handle any exceptions that may occur:
Task.Run(() =>
{
// Code that may throw an exception
}).ContinueWith((t) =>
{
// Log the error
File.AppendAllText("error.log", t.Exception.ToString());
// Display user-friendly message
MessageBox.Show("An error has occurred. Please try again later.");
}, TaskContinuationOptions.OnlyOnFaulted);
Conclusion
Error logging is an important aspect of any application, and is essential for debugging and fixing issues that may arise during the use of the application. By using try/catch
blocks, the Application.ThreadException
event, and a global exception handler, you can effectively log errors in a Windows Forms application written in C#. By following the best practices outlined in this blog post, you can ensure that all errors are logged and handled in an efficient and user-friendly manner.
It is important to note that error logging is just one aspect of a comprehensive error-handling strategy. Other considerations may include monitoring and alerting, handling errors in a production environment, and providing support to end users. By carefully planning and implementing a comprehensive error-handling strategy, you can ensure that your application is reliable and provides a good user experience. Make sure to reach out if you want to hear more about how elmah.io can help you.
elmah.io: Error logging and Uptime Monitoring for your web apps
This blog post is brought to you by elmah.io. elmah.io is error logging, uptime monitoring, deployment tracking, and service heartbeats for your .NET and JavaScript applications. Stop relying on your users to notify you when something is wrong or dig through hundreds of megabytes of log files spread across servers. With elmah.io, we store all of your log messages, notify you through popular channels like email, Slack, and Microsoft Teams, and help you fix errors fast.
See how we can help you monitor your website for crashes Monitor your website