Debugging System.FormatException when launching ASP.NET Core
I talked with a user the other day, experiencing a System.FormatException exception when trying to launch ASP.NET Core. While trying to help to figure out what went wrong, I realized that there can be a lot of different causes for this error in ASP.NET Core. This post is a summary of what I found out and actions to go through next time.
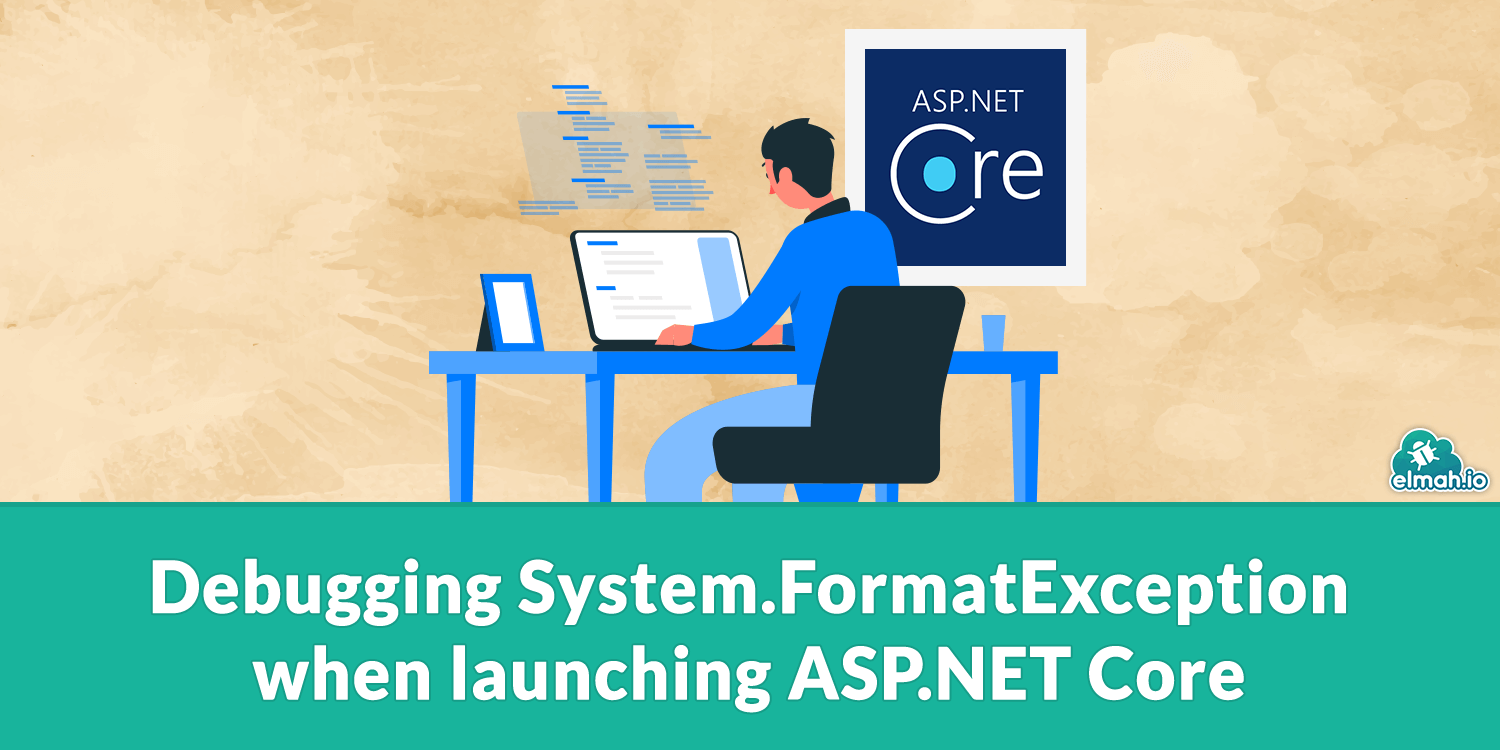
There are a number of problems that can cause a System.FormatException
during startup. If you haven't already, make sure to enable the developer exception page when running locally by including the following code in the Configure
method in the Startup.cs
file:
if (env.IsDevelopment())
{
app.UseDeveloperExceptionPage();
}
This will provide you with some additional information that can be helpful when needing to find the source of this error.
Here's a couple of variants of the exception:
JsonReaderException: The input does not contain any JSON tokens. Expected the input to start with a valid JSON token, when isFinalBlock is true. LineNumber: 0 | BytePositionInLine: 0.
JsonReaderException: 'd' is an invalid start of a property name. Expected a '"'. LineNumber: 1 | BytePositionInLine: 14.
JsonReaderException: ':' is invalid after a value. Expected either ',', '}', or ']'. LineNumber: 2 | BytePositionInLine: 14.
As you can see from the list, the FormatException
typically wrap a JsonReaderException
meaning that something is wrong with a JSON file. Here's a checklist to go through:
The steps in the following section should be executed for all of the following files:
appsettings.json
appsettings.*.json
(likeappsettings.development.json
)secrets.json
global.json
launchSettings.json
- Any custom
.json
files. ASP.NET Core supports including additional configuration through custom JSON files. Make sure to validate those as well.
And the steps to execute:
- Open the
appsettings.json
file and validate that the file contains any data. An empty file will cause aFormatException
. If you don't want any config in the file, as a minimum include{}
. - Verify that the JSON content is syntactically correct. You can use the built-in validation support in Visual Studio or an external validator like this one: JSON Formatter and Validator.
Also, you can consider validating that the content of the appsettings.*.json
files is valid using this Appsettings.json Validator. Invalid content usually doesn't cause a FormatException
but better safe than sorry.
elmah.io: Error logging and Uptime Monitoring for your web apps
This blog post is brought to you by elmah.io. elmah.io is error logging, uptime monitoring, deployment tracking, and service heartbeats for your .NET and JavaScript applications. Stop relying on your users to notify you when something is wrong or dig through hundreds of megabytes of log files spread across servers. With elmah.io, we store all of your log messages, notify you through popular channels like email, Slack, and Microsoft Teams, and help you fix errors fast.
See how we can help you monitor your website for crashes Monitor your website